Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
Today, I’m going to show How do I check if the first character of a string is a specific character in Javascript, here I will use the javascript string charAt()
method to check if the first character of a string is a specific character or not.
What is a charAt()
method?
The String object’s charAt()
method returns a new string consisting of the single UTF-16 code unit located at the specified offset into the string.
Let’s start the today’s tutorial How do you check if the first character of a string is a specific character in JavaScript?
Let’s understand how we can do it
- Take sample string variable
- Use the
charAt()
method with index 0 to get first character - Use compare operator between the first character and specific character and console whether it’s a match or not
// Take sample string variable
const str = 'Hello world';
// Use the `charAt()` method with index 0 to get first character
let firstChar = str.charAt(0);
console.log("firstChar", firstChar);
// Use compare operator between the first character and specific character and console whether it's a match or not
// valid case example
if (firstChar == 'H') {
console.log("First character is our specific character");
} else {
console.log("First character isn't our specific character");
}
// invalid case example
if (firstChar == 'E') {
console.log("First character is our specific character");
} else {
console.log("First character isn't our specific character");
}
The above program is the simplest way to check string first character of a string is a specific character or not. let’s check the output.
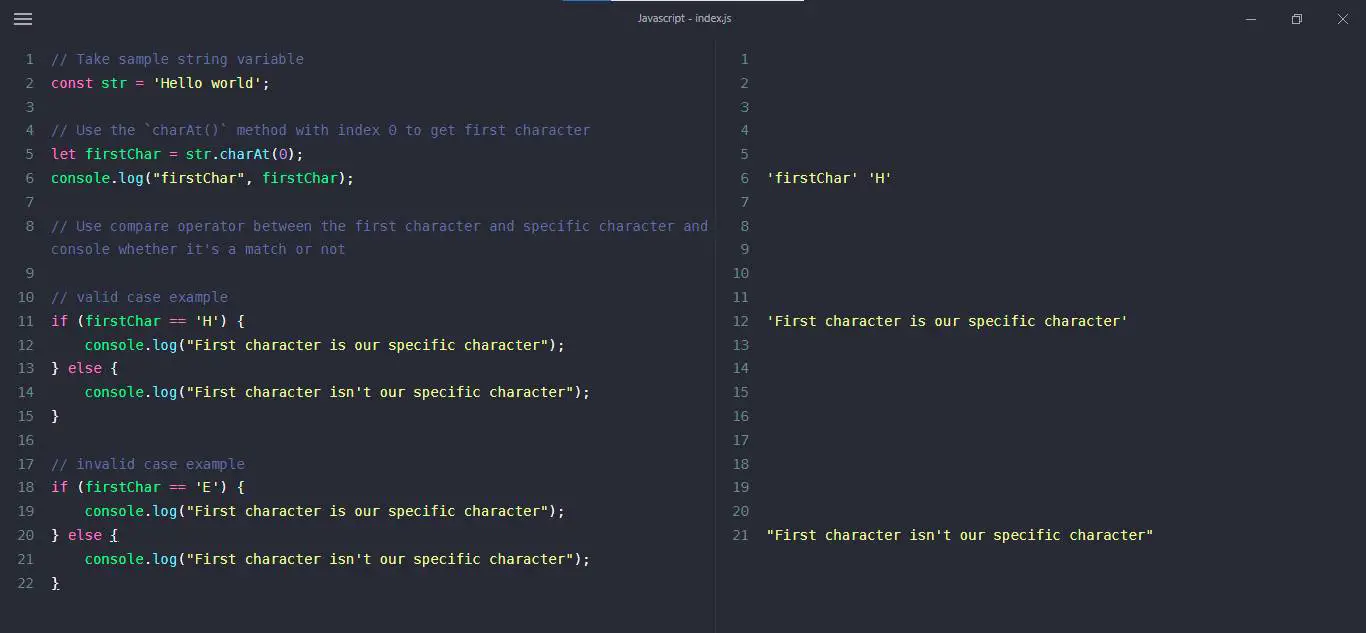
I hope it’s help you, All the best 👍.