Hi Friends š,
Welcome To Infinitbility! ā¤ļø
Today, Iām going to show you how do I find and remove elements from an array in javascript, here I will use the javascript findIndex()
method and some conditions to find the exact object in the array, and the splice()
method to remove a specific element from an array using element index value.
-
The
findIndex()
method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1, indicating that no element passed the test. -
The
splice()
method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place. To access part of an array without modifying it, see slice().
Well, letās start todayās topic How to find and remove elements from an array in javascript?
Before going to code we have below things.
First, we have any property value which we will use to find in an array.
The second is array š.
Okay, letās plan what we are going to do in code.
- Create a sample array variable.
- To get index, use
findIndex()
method - write condition to get the index of desire element
- remove an element from an array using an index value
// Create sample array of objects variable.
const domains = ["infinitbility", "aGuideHub", "SortoutCode"];
// To get index, use `findIndex()` method
// write condition to get index of desire element
let index = domains.findIndex(e => e == "aGuideHub");
console.log("index", index);
// Expected 'index' 1
// remove element from array using index value
domains.splice(index, 1)
console.log("domains", domains);
// 'domains' [ 'infinitbility', 'SortoutCode' ]
In the above example, Iām using findIndex()
and comparing every element to get desired element index from the array.
Here, as per expectation, it should show 'domains' [ 'infinitbility', 'SortoutCode' ]
, letās check the output.
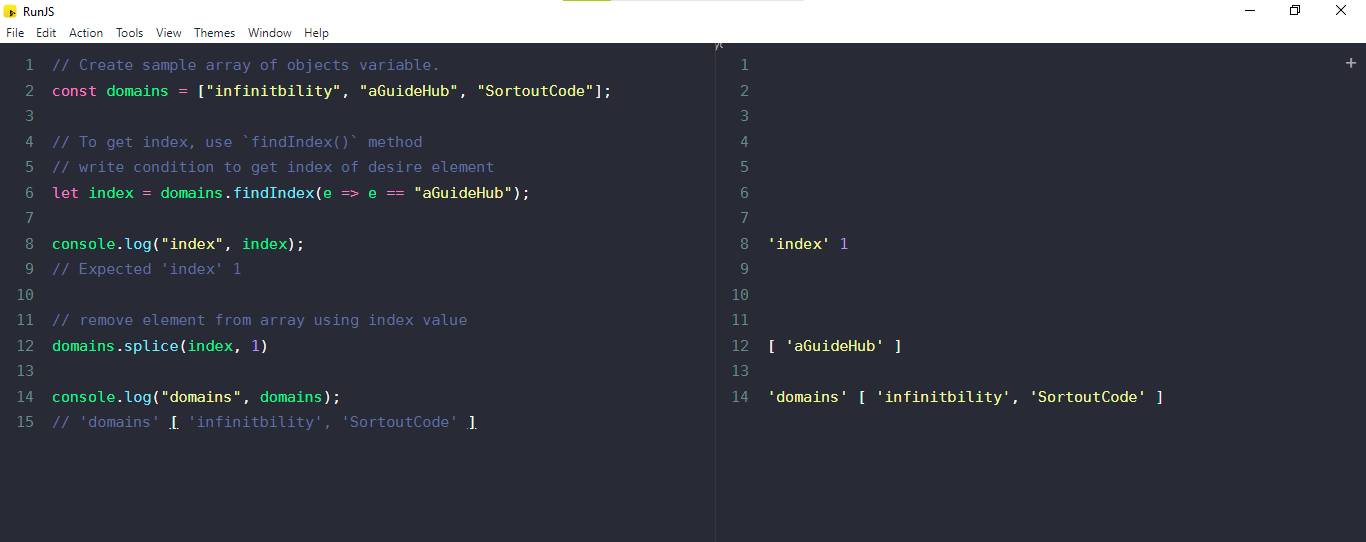
I hope itās help you, All the best š.