Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
To compare two dates in moment js, The momentjs provide isBefore()
, isSame()
, isAfter()
, isSameOrBefore()
, isSameOrAfter()
, and isBetween()
to compare dates as you want.
Today, I’m going to show How do I compare two dates in moment js, here I will use the momentjs above-mentioned functions with example to compare dates.
Let’s start the today’s tutorial How do you compare two dates in moment js?
Table of content
- Installation
- Check if a date is before another date
- Check if a date is the same as another date
- Check if a date is after another date
- Check if a date is the same or before another date
- Check if a date is the same or after another date
- Check if a date is between other dates
- Examples in reactjs
Installation
Use the below installation command as per your package manager, moment support npm, Yarn, NuGet, spm, and meteor.
npm install moment --save # npm
yarn add moment # Yarn
Install-Package Moment.js # NuGet
spm install moment --save # spm
meteor add momentjs:moment # meteor
Check if a date is before another date
The momentjs provide isBefore()
method to check date is before another date or not if its return is true
means the date is before another date.
moment('2010-10-20').isBefore('2010-10-21'); // true
You can specify what you are checking like year
, month
, week
, isoWeek
, day
, hour
, minute
, and second
using isBefore()
second parameter like below example.
moment('2010-10-20').isBefore('2010-12-31', 'year'); // false
moment('2010-10-20').isBefore('2011-01-01', 'year'); // true
Check if a date is same another date
The momentjs provide the isSame()
method to check date is the same as another date or not, if it returns true
means the date is the same as another date.
moment('2010-10-20').isSame('2010-10-20'); // true
You can specify what you are checking like year
, month
, week
, isoWeek
, day
, hour
, minute
, and second
using isSame()
second parameter like below example.
moment('2010-10-20').isSame('2009-12-31', 'year'); // false
moment('2010-10-20').isSame('2010-01-01', 'year'); // true
moment('2010-10-20').isSame('2010-12-31', 'year'); // true
moment('2010-10-20').isSame('2011-01-01', 'year'); // false
When including a second parameter, it will match all units equal or larger. Passing in month
will check month
and year
. Passing in day
will check day
, month
, and year
.
moment('2010-01-01').isSame('2011-01-01', 'month'); // false, different year
moment('2010-01-01').isSame('2010-02-01', 'day'); // false, different month
Check if a date is after another date
The momentjs provide the isAfter()
method to check date is after another date or not, if its return is true
means the date is after another date.
moment('2010-10-20').isAfter('2010-10-19'); // true
You can specify what you are checking like year
, month
, week
, isoWeek
, day
, hour
, minute
, and second
using isAfter()
second parameter like below example.
moment('2010-10-20').isAfter('2010-01-01', 'year'); // false
moment('2010-10-20').isAfter('2009-12-31', 'year'); // true
Check if a date is same or before another date
The momentjs provide the isSameOrBefore()
method to check date is the same or before another date or not if its return true
means the date is the same or before another date.
moment('2010-10-20').isSameOrBefore('2010-10-21'); // true
moment('2010-10-20').isSameOrBefore('2010-10-20'); // true
moment('2010-10-20').isSameOrBefore('2010-10-19'); // false
You can specify what you are checking like year
, month
, week
, isoWeek
, day
, hour
, minute
, and second
using isSameOrBefore()
second parameter like below example.
moment('2010-10-20').isSameOrBefore('2009-12-31', 'year'); // false
moment('2010-10-20').isSameOrBefore('2010-12-31', 'year'); // true
moment('2010-10-20').isSameOrBefore('2011-01-01', 'year'); // true
Check if a date is same or after another date
The momentjs provide the isSameOrAfter()
method to check date is the same or after another date or not if it returns true
means the date is the same or after another date.
moment('2010-10-20').isSameOrAfter('2010-10-19'); // true
moment('2010-10-20').isSameOrAfter('2010-10-20'); // true
moment('2010-10-20').isSameOrAfter('2010-10-21'); // false
You can specify what you are checking like year
, month
, week
, isoWeek
, day
, hour
, minute
, and second
using isSameOrAfter()
second parameter like below example.
moment('2010-10-20').isSameOrAfter('2011-12-31', 'year'); // false
moment('2010-10-20').isSameOrAfter('2010-01-01', 'year'); // true
moment('2010-10-20').isSameOrAfter('2009-12-31', 'year'); // true
Check if a date is between another dates
The momentjs provide the isBetween()
method to check date is between another date or not if it returns true
means the date is between another date.
moment('2010-10-20').isBetween('2010-10-19', '2010-10-25'); // true
moment('2010-10-20').isBetween('2010-10-25', '2010-10-19'); // false
You can specify what you are checking like year
, month
, week
, isoWeek
, day
, hour
, minute
, and second
using isBetween()
third parameter like below example.
moment('2010-10-20').isBetween('2010-01-01', '2012-01-01', 'year'); // false
moment('2010-10-20').isBetween('2009-12-31', '2012-01-01', 'year'); // true
Example in reactjs
In the following example, we are going to do, Put all the above function examples in a function and check their output.
let’s write the code.
import moment from "moment";
function consoleTheMoment() {
console.log("------- Is Before -------");
console.log("moment('2010-10-20').isBefore('2010-10-21')", moment('2010-10-20').isBefore('2010-10-21'))
console.log("------- Is Same -------");
console.log("moment('2010-10-20').isSame('2010-10-20')", moment('2010-10-20').isSame('2010-10-20'))
console.log("------- Is After -------");
console.log("moment('2010-10-20').isAfter('2010-10-19')", moment('2010-10-20').isAfter('2010-10-19'))
console.log("------- Is same or before -------");
console.log("moment('2010-10-20').isSameOrBefore('2010-10-21')", moment('2010-10-20').isSameOrBefore('2010-10-21'))
console.log("moment('2010-10-20').isSameOrBefore('2010-10-20')", moment('2010-10-20').isSameOrBefore('2010-10-20'))
console.log("moment('2010-10-20').isSameOrBefore('2010-10-19')", moment('2010-10-20').isSameOrBefore('2010-10-19'))
console.log("------- Is same or after -------");
console.log("moment('2010-10-20').isSameOrAfter('2010-10-19')", moment('2010-10-20').isSameOrAfter('2010-10-19'))
console.log("moment('2010-10-20').isSameOrAfter('2010-10-20')", moment('2010-10-20').isSameOrAfter('2010-10-20'))
console.log("moment('2010-10-20').isSameOrAfter('2010-10-21')", moment('2010-10-20').isSameOrAfter('2010-10-21'))
console.log("------- Is between -------");
console.log("moment('2010-10-20').isBetween('2010-10-19', '2010-10-25')", moment('2010-10-20').isBetween('2010-10-19', '2010-10-25'))
console.log("moment('2010-10-20').isBetween('2010-10-25', '2010-10-19')", moment('2010-10-20').isBetween('2010-10-25', '2010-10-19'))
}
function App() {
consoleTheMoment()
return (
<div>
{moment().format("hh:mm:ss")}
</div>
);
}
export default App;
In the above program, we call moment comparison methods in simple function to compare dates. let’s check the output.
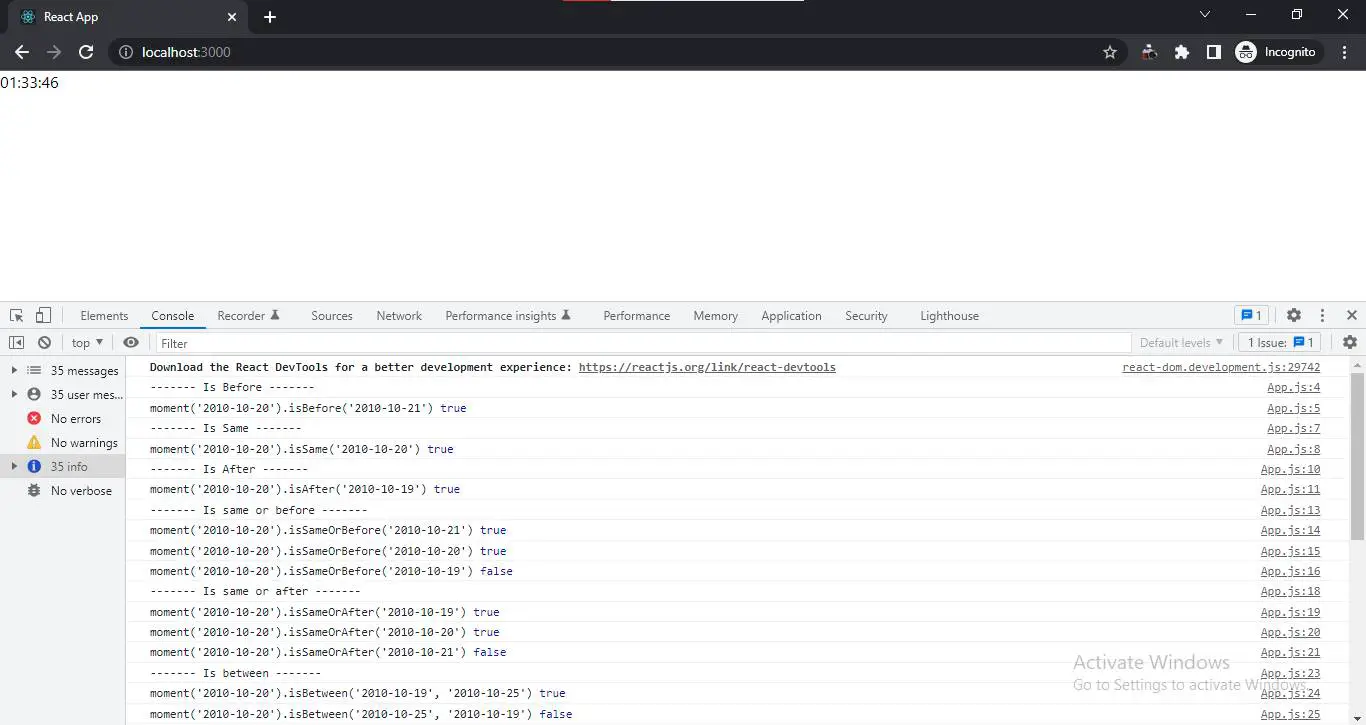
I hope it’s help you, All the best 👍.