Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
To convert the object to an array in react native, just use Object.entries()
to iterate the object and use the map()
method to create an array of objects.
Basically, the Object.entries()
method makes object key-value pair to an array of array, where every array has a key and value as an element of the array. like the following example.
const x = { foo: 11, bar: 42 };
console.log(Object.entries(x));
// Output
// [['foo', 11], ['bar', 42]]
After that, we will use the map()
method to create desired structure of the array.
Today, I’m going to show you How do i convert a JSON object to an array in react native, as above mentioned here, I’m going to use the Object.entries()
and map()
methods to convert object to array.
Let’s start today’s tutorial how do you convert JSON object to array in react native?
In the above example, we will do
- Create a sample object variable.
- Use
Object.entries()
andmap()
method - Print the Output on the screen
import React, { useState, useEffect } from 'react';
import { Text, View, StyleSheet } from 'react-native';
export default function App() {
const [domains, setDomains] = useState('');
useEffect(() => {
let domains = { id: 1, name: 'infinitbility.com' };
const result = Object.entries(domains).map(([key, val]) => ({[key]: val}));
console.log(result);
setDomains(JSON.stringify(result));
}, []);
return (
<View style={styles.container}>
{domains}
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
backgroundColor: '#ecf0f1',
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 18,
fontWeight: 'bold',
textAlign: 'center',
},
});
As above mentioned, we are taken the example of the domain object and converted it into an array of objects.
Let’s check the output.
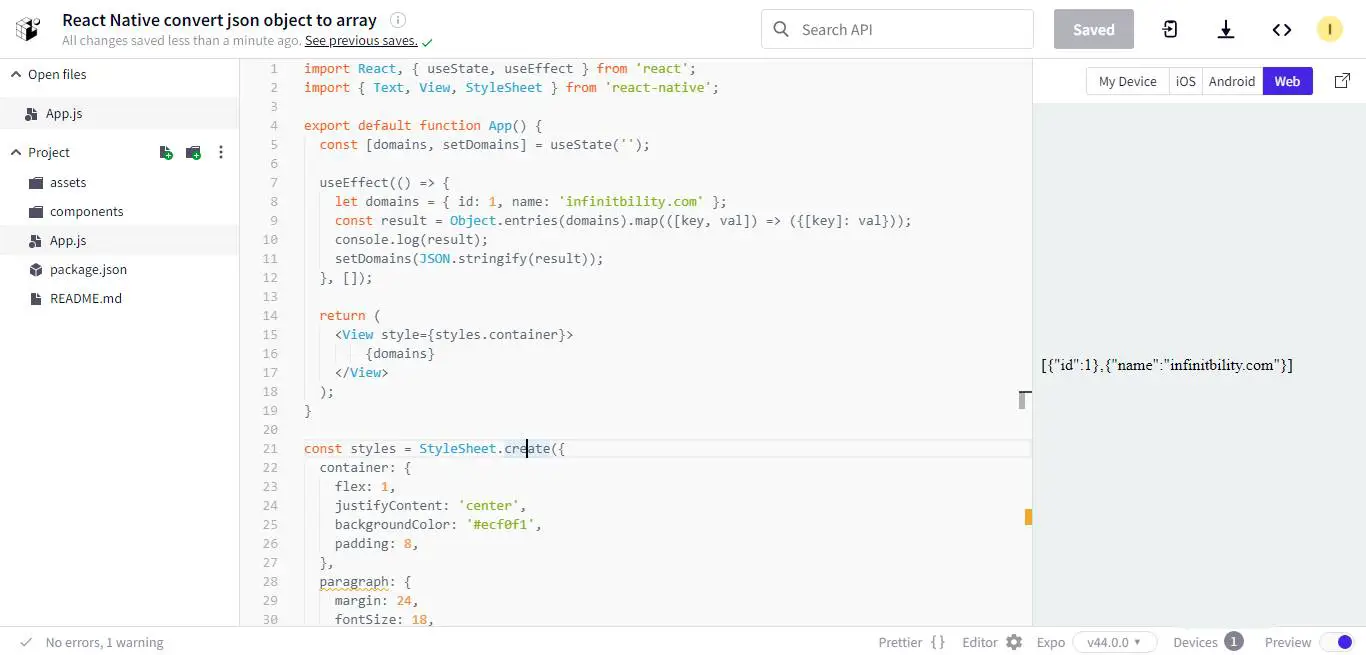
I hope it’s help you, All the best 👍.