Hello Friends đź‘‹,
Welcome To Infinitbility! ❤️
This tutorial will help you to detect is battery charging in ios, android, windows and web, here we will use react-native-device-info ( https://github.com/react-native-device-info/react-native-device-info ) for detection currently battery charging or not.
Let’s start today tutorial How to detect battery charging or not in react native
Introduction
React Native device info provide isBatteryCharging()
function to check battery charging or not it will return only true
or false
. isBatteryCharging()
function work in ios, android, windows, and web also.
Installation
- Go to your project directory and install
react-native-device-info
package usingnpm
.
npm install --save react-native-device-info
- Link
react-native-device-info
package if your react native version less then0.63
.
npx react-native link react-native-device-info
Syntax
DeviceInfo.isBatteryCharging()
function usages syntax.
import DeviceInfo from 'react-native-device-info';
DeviceInfo.isBatteryCharging().then((isCharging) => {
if (!isCharging) {
// ...
}
});
Example
In Below Example, we will isBatteryCharging()
value on constant and show in application.
React Native Device Info isBatteryCharging example
import React, { Component } from 'react';
import {
StyleSheet,
View,
Text,
SafeAreaView,
} from 'react-native';
import DeviceInfo from 'react-native-device-info';
class App extends Component {
constructor(props) {
super(props);
this.state = {
isBatteryCharging: false
}
}
componentDidMount(){
DeviceInfo.isBatteryCharging().then((isCharging) => {
if (isCharging) {
this.setState({isBatteryCharging: true});
}
});
}
render() {
const { isBatteryCharging } = this.state;
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={styles.container}>
<Text>Device is charging</Text>
<Text>{`${isBatteryCharging}`}</Text>
</View>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
marginTop: 20,
backgroundColor: '#ffffff',
},
});
export default App;
Output
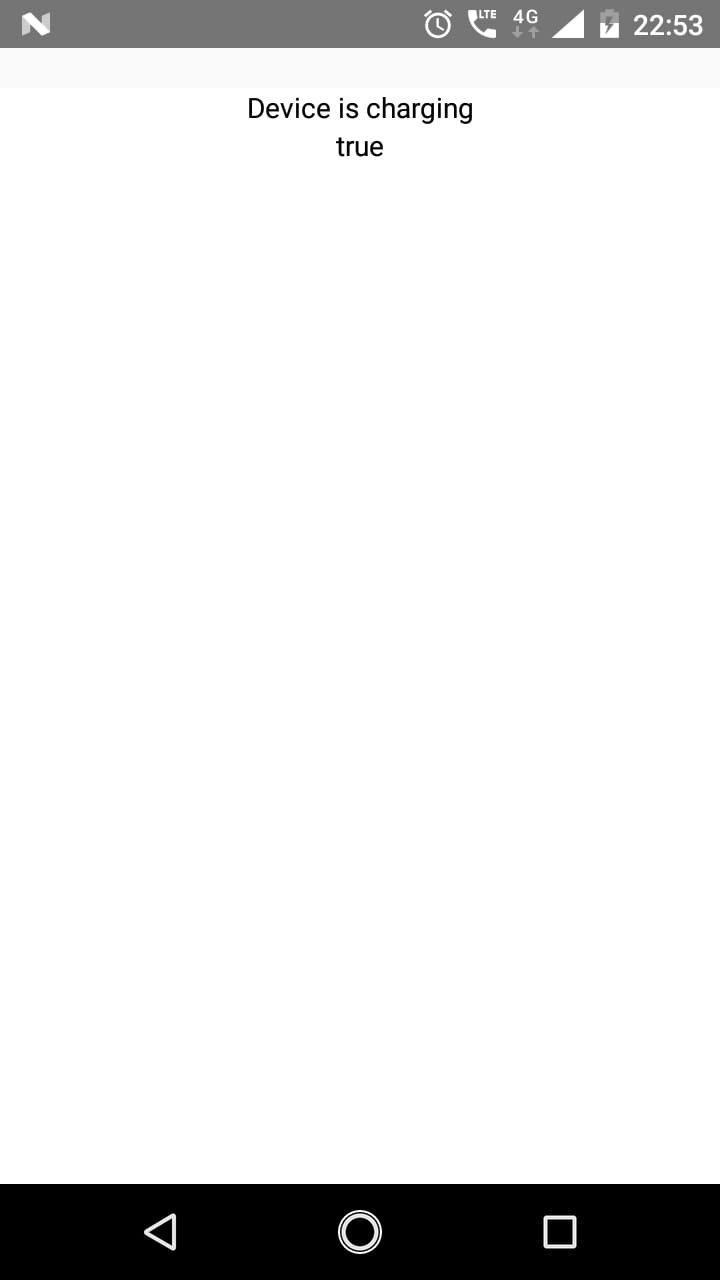
Thanks for Reading…