Hello Friends đź‘‹,
Welcome To Infinitbility! ❤️
This tutorial will help you to detect device have notch or not in ios, android, and windows, here we will use react-native-device-info ( https://github.com/react-native-device-info/react-native-device-info ) for detection device have notch or not.
Let’s start today tutorial How to detect notch in react native
What is notch?
Notch at phone is a small cut-out, which is placed at the top part of the your screen display. A notch display smartphone offers more screen display at your smartphone. You can also get battery, date & time, network signals information at the top part of your smartphone.
Introduction
React Native device info provide hasNotch()
function to check device have notch or not it will return only true
or false
. hasNotch()
function work in ios, android, and windows.
Installation
- Go to your project directory and install
react-native-device-info
package usingnpm
.
npm install --save react-native-device-info
- Link
react-native-device-info
package if your react native version less then0.63
.
npx react-native link react-native-device-info
Syntax
DeviceInfo.hasNotch()
function usages syntax.
import DeviceInfo from 'react-native-device-info';
let hasNotch = DeviceInfo.hasNotch();
// true
Example
In Below Example, we will hasNotch()
value on constant and show in application.
React Native Device Info hasNotch example
import React, { Component } from 'react';
import {
StyleSheet,
View,
Text,
SafeAreaView,
} from 'react-native';
import DeviceInfo from 'react-native-device-info';
class App extends Component {
constructor(props) {
super(props);
this.state = {
hasNotch: false
}
}
componentDidMount(){
let hasNotch = DeviceInfo.hasNotch();
this.setState({hasNotch: hasNotch});
}
render() {
const { hasNotch } = this.state;
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={styles.container}>
<Text>Device have notch</Text>
<Text>{`${hasNotch}`}</Text>
</View>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
marginTop: 20,
backgroundColor: '#ffffff',
},
});
export default App;
Output
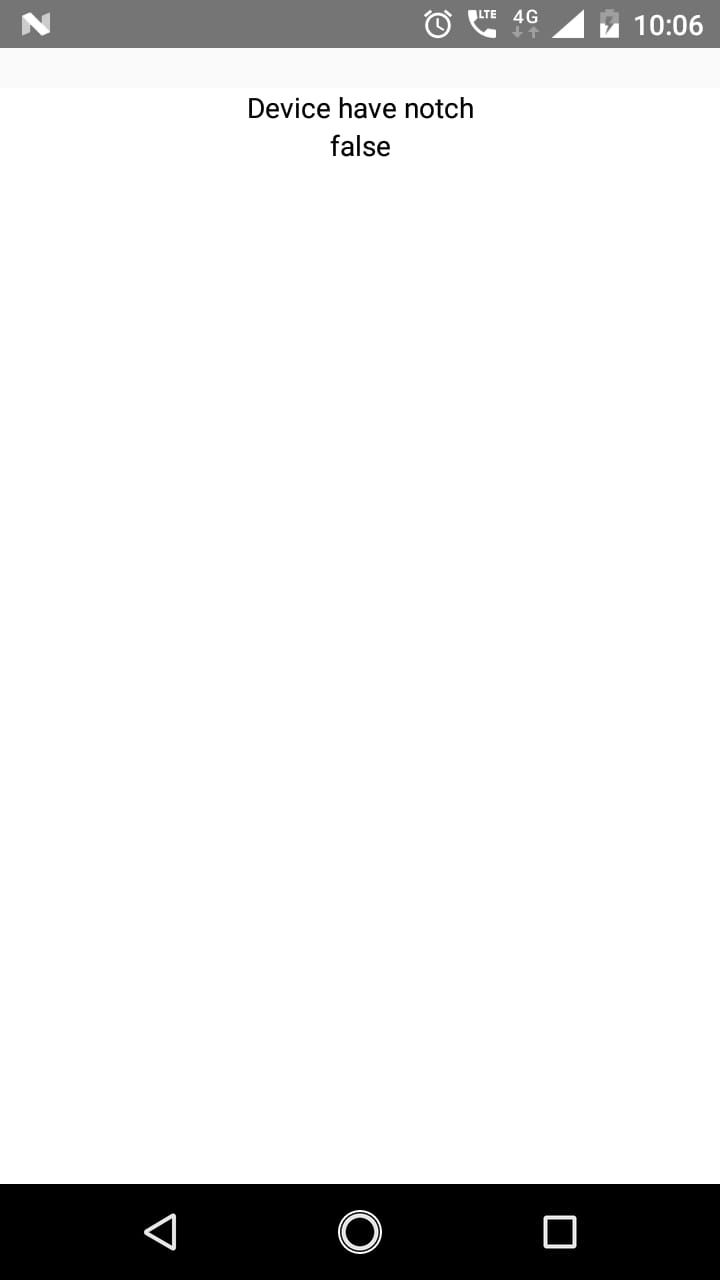
Looking for get statusBarHeight?
I have found code that is I think the best way to get height in android and ios.
StatusBarHeight.js
import { Dimensions, Platform, StatusBar } from 'react-native';
const X_WIDTH = 375;
const X_HEIGHT = 812;
const XSMAX_WIDTH = 414;
const XSMAX_HEIGHT = 896;
const { height, width } = Dimensions.get('window');
export const isIPhoneX = () => Platform.OS === 'ios' && !Platform.isPad && !Platform.isTVOS
? width === X_WIDTH && height === X_HEIGHT || width === XSMAX_WIDTH && height === XSMAX_HEIGHT
: false;
export const StatusBarHeight = Platform.select({
ios: isIPhoneX() ? 44 : 20,
android: StatusBar.currentHeight,
default: 0
})
Then use it directly when imported:
import { StatusBarHeight } from './StatusBarHeight.js'
height: StatusBarHeight
Thanks for Reading…