Hello Friends đź‘‹,
Welcome To Infinitbility! ❤️
This tutorial will help you to filter the array of objects by another array of objects with sample code of EcmaScript 6 and the old version also.
Lots of times we need to filter an array of objects by another array of objects and here we write multiple loops with push do a task which is ok for older versions, but now we have a better way to do it.
The following example will work if you are using EcmaScript 6 or the upper version, here we have used EcmaScript 6 filter()
and some()
methods.
-
The
filter()
method creates a new array with all elements that pass the test implemented by the provided function. -
The
some()
method tests whether at least one element in the array passes the test implemented by the provided function. It returns true if, in the array, it finds an element for which the provided function returns true; otherwise it returns false. It doesn’t modify the array.
const myArray = [
{ userid: "100", projectid: "10", rowid: "0" },
{ userid: "101", projectid: "11", rowid: "1"},
{ userid: "102", projectid: "12", rowid: "2" },
{ userid: "103", projectid: "13", rowid: "3" },
{ userid: "101", projectid: "10", rowid: "4" }
];
const myFilter = [
{ userid: "101", projectid: "11" },
{ userid: "102", projectid: "12" },
{ userid: "103", projectid: "11"}
];
const myArrayFiltered = myArray.filter((el) => {
return myFilter.some((f) => {
return f.userid === el.userid && f.projectid === el.projectid;
});
});
console.log(myArrayFiltered);
Output
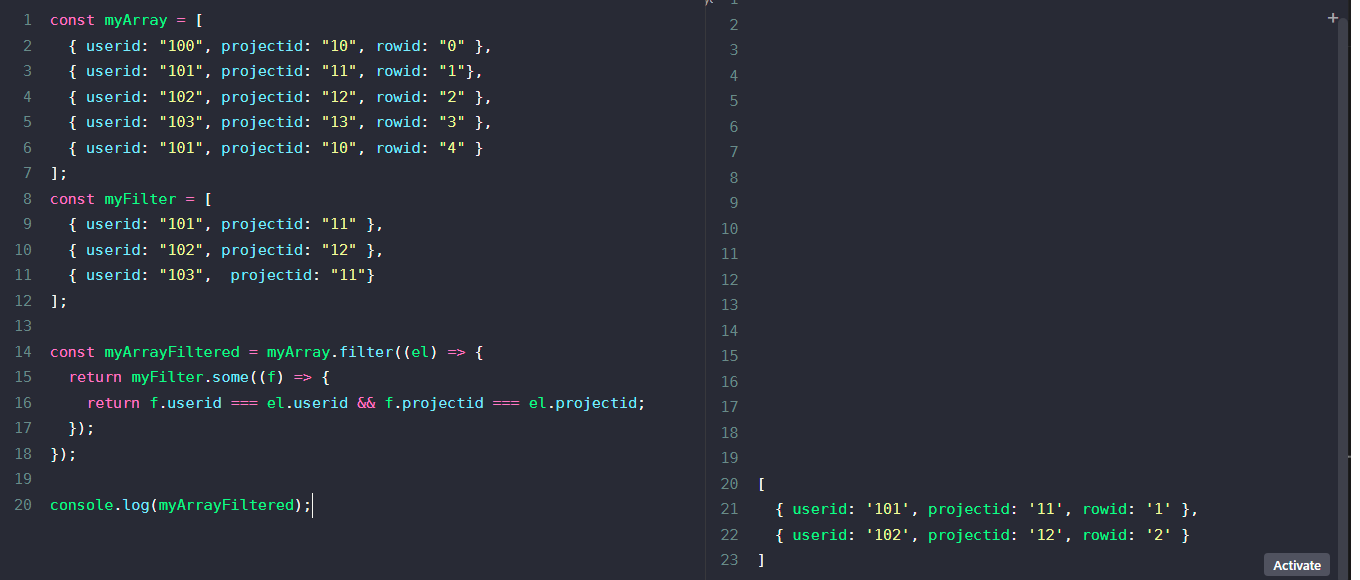
Are you using the old version of EcmaScript follow the below example it will surely work for you because it’s isn’t using any EcmaScript 6.
Here, we are using multiple loops with the push method to create a filtered separate array.
const myArray = [
{ userid: "100", projectid: "10", rowid: "0" },
{ userid: "101", projectid: "11", rowid: "1"},
{ userid: "102", projectid: "12", rowid: "2" },
{ userid: "103", projectid: "13", rowid: "3" },
{ userid: "101", projectid: "10", rowid: "4" }
];
const myFilter = [
{ userid: "101", projectid: "11" },
{ userid: "102", projectid: "12" },
{ userid: "103", projectid: "11"}
];
let filtered = [];
for(let arr in myArray){
for(let filter in myFilter){
if(myArray[arr].userid == myFilter[filter].userid && myArray[arr].projectid == myFilter[filter].projectid){
filtered.push(myArray[arr]);
}
}
}
console.log(filtered);
Output
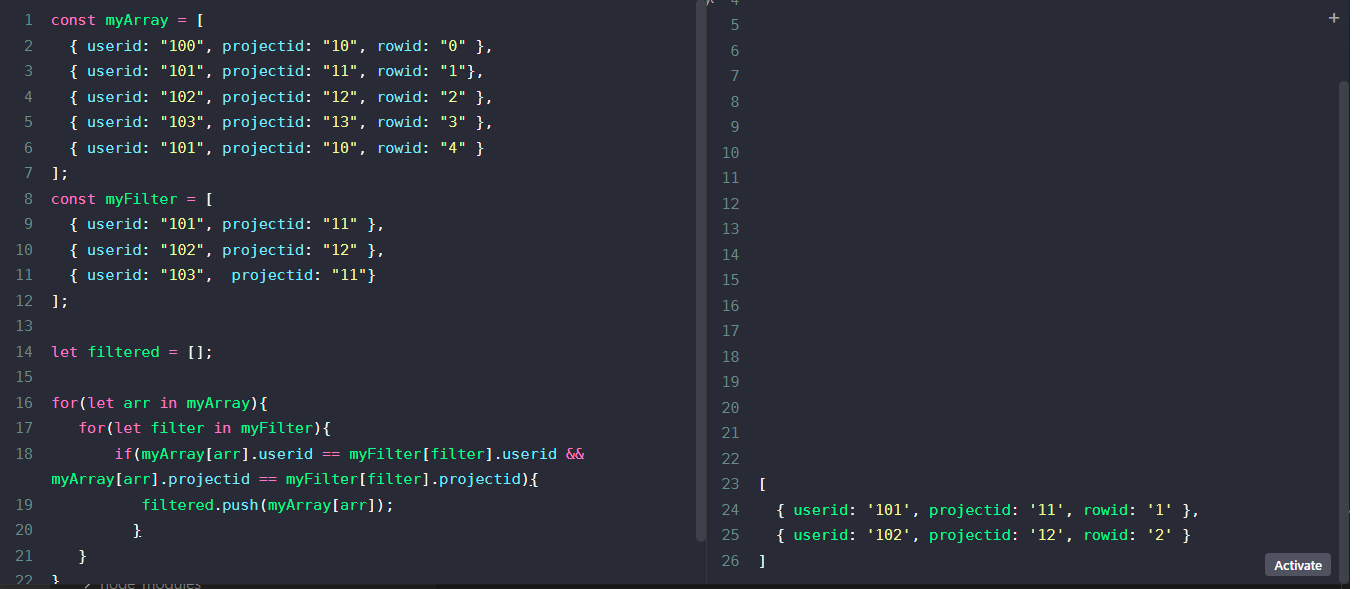
Thanks for reading…