Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
Today, we will see how can we get all values from nested JSON objects, here we will use a custom function which will return the list of values available in a nested JSON object.
So, we will create a function that navigates every possible object’s key and value and add values in an array that returns at the end of recursive loops.
Well, let’s create a function that returns the values of nested objects.
const getNestedValues = (data, values) => {
if(!(data instanceof Array) && typeof data == 'object'){
Object.values(data).forEach( value => {
if(typeof value === 'object' && !(value instanceof Array)){
getNestedValues(value, values);
} else {
values.push(value);
}
});
}
return values
}
In javascript when we check typeof of the array it will return i.e we use instanceof Array
to handle array types of object.
Let’s take an example below the nested object.
const data = {
person: {
male: {
name: "infinitbility"
},
female: {
name: "aguidehub"
}
}
};
const getNestedValues = (data, values) => {
if(!(data instanceof Array) && typeof data == 'object'){
Object.values(data).forEach( value => {
if(typeof value === 'object' && !(value instanceof Array)){
getNestedValues(value, values);
} else {
values.push(value);
}
});
}
return values
}
getNestedValues(data, []);
// [ 'infinitbility', 'aguidehub' ]
When you run the above code, you will get [ 'infinitbility', 'aguidehub' ]
let’s check the output.
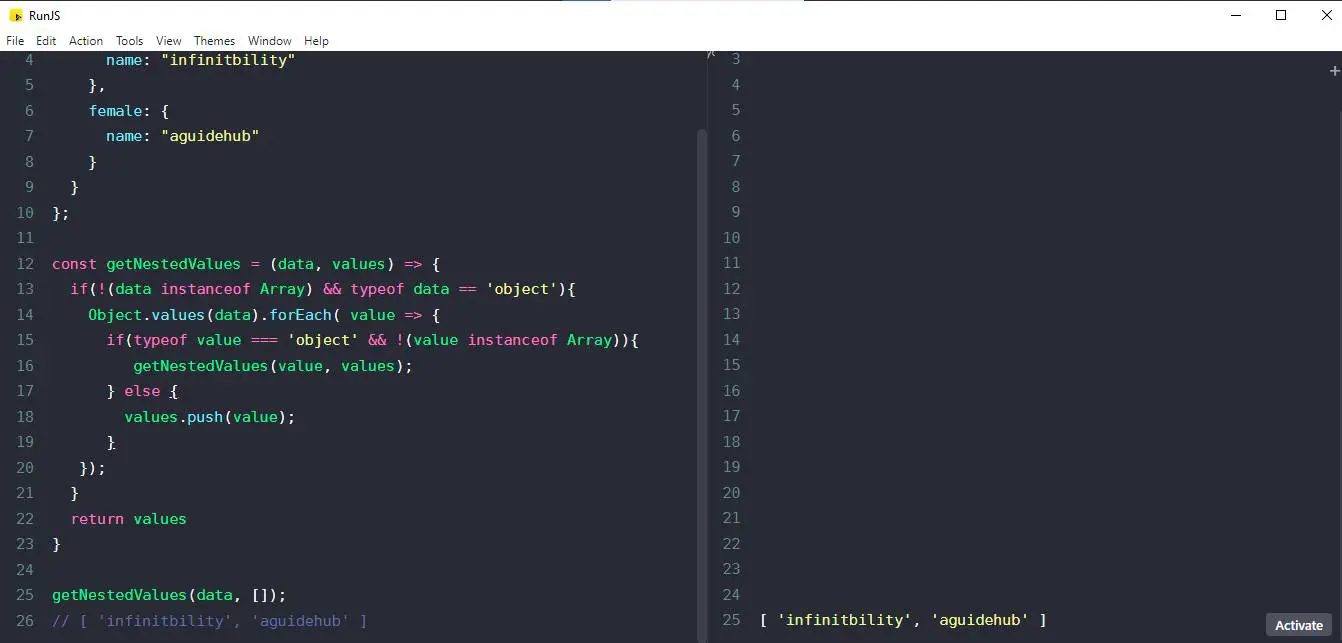
Want to reomove duplicate, read below tutorial.
https://infinitbility.com/how-to-remove-duplicates-from-array-of-strings-in-javascript
All the best 👍.