Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
To get length of json object in javascript, just use the Object.keys()
method with the length property. it will return the length of the object.
Today, we will learn How to get a count of a JSON object in javascript, here we will use the keys()
method and array length to get a count of a JSON object.
Basically, The keys()
method creates an array of object keys, and when we get an array, we will use simple array length syntax to get a count of objects.
Well, let’s start today’s tutorial How to get a count of JSON objects in javascript?
Here, we will do
- create a sample object variable
- use
Object.keys()
method to get an array - use
.length
to get the number of keys
// create sample object variable
let sampleObject = {
id: 1,
name: "infinitbility",
domain: "infinitbility.com",
email: "[email protected]"
};
// convert keys to array
let sampleObjectKeys = Object.keys(sampleObject);
console.log("sampleObjectKeys", sampleObjectKeys)
// print the number of keys
console.log("number of keys", sampleObjectKeys.length);
we can also write in one line like the below example.
console.log(Object.keys(sampleObject).length);
let’s run it, it should show the 4
size of the object.
Output
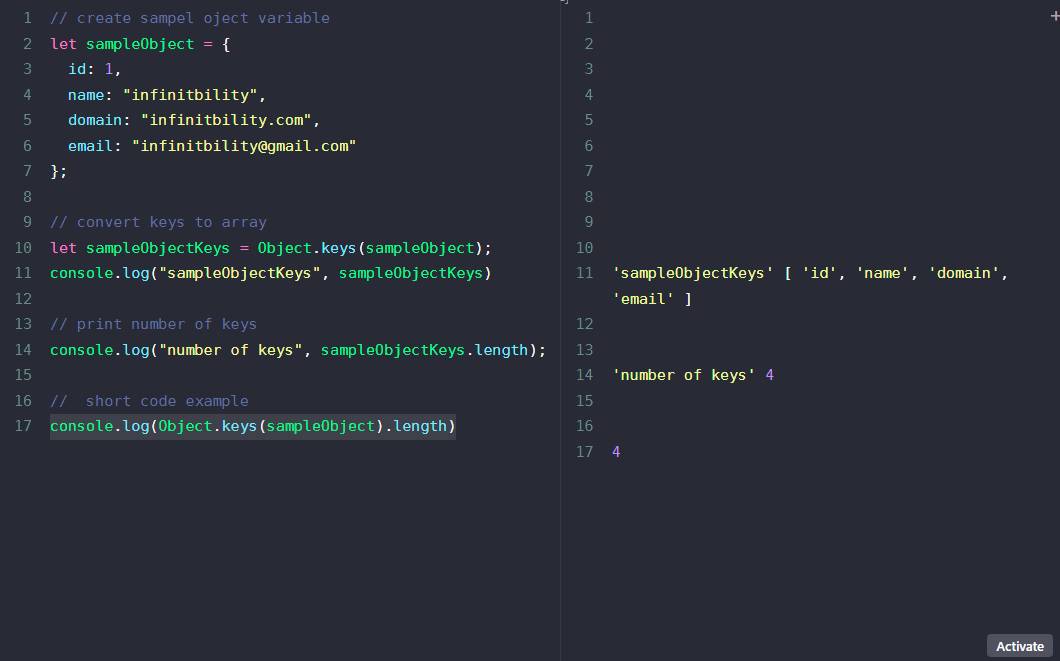
Handle even object contain symbol keys
The Objects can have symbolic properties that can not be returned via Object.key method. So the answer would be incomplete without mentioning them.
Symbol type was added to the language to create unique identifiers for object properties. The main benefit of the Symbol type is the prevention of overwrites.
Object.keys
or Object.getOwnPropertyNames
does not work for symbolic properties. To return them you need to use Object.getOwnPropertySymbols
.
var person = {
[Symbol('name')]: 'John Doe',
[Symbol('age')]: 33,
"occupation": "Programmer"
};
const propOwn = Object.getOwnPropertyNames(person);
console.log(propOwn.length); // 1
let propSymb = Object.getOwnPropertySymbols(person);
console.log(propSymb.length); // 2
Output
All the best 👍.