Hello Friends đź‘‹,
Welcome To Infinitbility! ❤️
this tutorial will help you to get month name in react native, here we learn to show month name using javascript current date or using moment.js package.
Let’s start today tutorial How to get current month name in react native?
Show month name using months constants
javascript date object provide getMonth()
function to get month index number and now we will use this index in months array and show Month name based on date.
// show month using javascript code
const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
const d = new Date();
let monthName = months[d.getMonth()];
Show month name using moment
For using moment, first we have to install moment package in our react native project. use Below command to install package
npm install moment --save
Moment provide format functinality to convert current date to show month name.
moment().format("MMMM");
Show Month name example
On Below example, i have store both ways to get month in state and showing in screen.
import React, { Component } from 'react';
import {
StyleSheet,
View,
Text,
SafeAreaView,
} from 'react-native';
import moment from 'moment';
class App extends Component {
constructor(props) {
super(props);
this.state = {
monthName: null,
momentMonthName: null
}
}
componentDidMount(){
// show month using javascript code
const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
const d = new Date();
let monthName = months[d.getMonth()];
this.setState({monthName: monthName});
// show month name using moment
let momentMonthName = moment().format("MMMM");
this.setState({momentMonthName: momentMonthName});
}
render() {
const { monthName, momentMonthName } = this.state;
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={styles.container}>
<Text>Showing month name using javascript code</Text>
<Text>{`${monthName}`}</Text>
<Text>Showing month name using moment</Text>
<Text>{`${momentMonthName}`}</Text>
</View>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
marginTop: 20,
backgroundColor: '#ffffff',
},
});
export default App;
Output
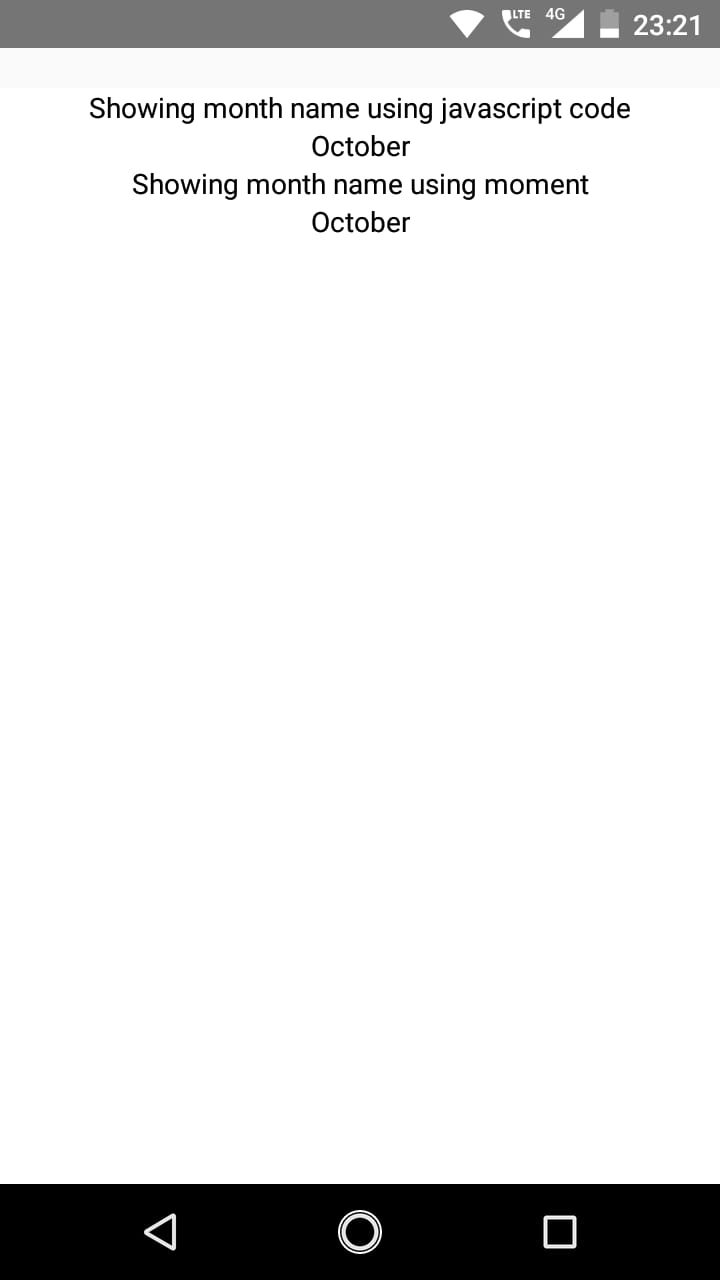
Thanks for Reading…