Hello Friends đź‘‹,
Welcome To Infinitbility! ❤️
This tutorial will help you to get ip address and ipv4address in react native, and in this tutorial we will use React native network info to get ipaddress of device.
Let’s start today tutorial How to get ip address in react native?
Introduction
React native network info provide getIPAddress()
method to get ip address of device, it will simply return data in string data type, and one more thing getIPAddress()
available on both Platform android, and iOS.
Installation
- Go to your project directory and install
react-native-network-info
package usingnpm
.
npm install --save react-native-network-info
- Link
react-native-network-info
package if your react native version less then0.63
.
npx react-native link react-native-network-info
Syntax
DeviceInfo.getIPAddress()
function usages syntax.
import {NetworkInfo} from 'react-native-network-info';
// Get Local IP
NetworkInfo.getIPAddress().then(ipAddress => {
console.log(ipAddress);
});
NetworkInfo.getIPV4Address().then(ipv4Address => {
console.log(ipv4Address);
});
Example
In Below Example, we will store getIPAddress()
value on state and show in application.
React Native Network Info getIPAddress example
import React, { Component } from 'react';
import {
StyleSheet,
View,
Text,
SafeAreaView,
} from 'react-native';
import {NetworkInfo} from 'react-native-network-info';
class App extends Component {
constructor(props) {
super(props);
this.state = {
ipAddress: null,
ipv4Address: null
}
}
componentDidMount(){
NetworkInfo.getIPAddress().then(ipAddress => {
this.setState({ipAddress: ipAddress});
});
NetworkInfo.getIPV4Address().then(ipv4Address => {
this.setState({ipv4Address: ipv4Address});
});
}
render() {
const { ipv4Address, ipAddress } = this.state;
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={styles.container}>
<Text>Your device ipAddress is </Text>
<Text>{`${ipAddress}`}</Text>
<Text>Your device ipv4Address is </Text>
<Text>{`${ipv4Address}`}</Text>
</View>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
marginTop: 20,
backgroundColor: '#ffffff',
},
});
export default App;
Output
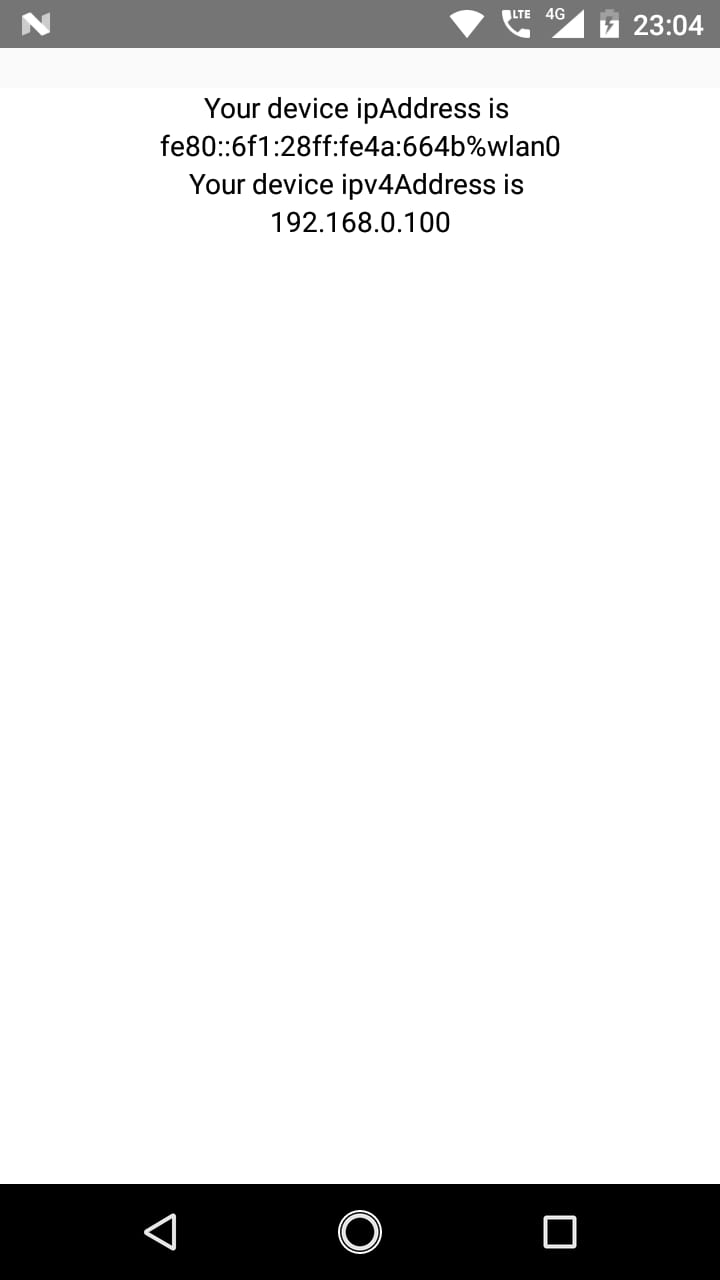
Thanks for Reading…