Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
To get keys and values from a JSON array of objects in react native, use the map()
to iterate and Object.keys()
to get the keys of an object using both methods we are able to iterate and use keys and values of JSON array of objects.
Today, I’m going to show How do I get keys and values from a JSON array of objects in react native, here I will use the javascript map()
and Object.keys()
method to get keys and values from JSON array of objects.
Let’s start today’s tutorial How do you get keys and values from a JSON array of objects in react native?
Example in React Native
In the following example, we are going to do
- Take example JSON array of objects
- apply the
map()
method to iterate the array. - apply
Object.keys()
to get the key and value both from the object - Print on react native app screen
import React, { useState, useEffect } from 'react';
import { Text, View, StyleSheet } from 'react-native';
export default function App() {
const [domains, setDomains] = useState([]);
useEffect(() => {
let domains = [
{ id: 1, name: 'infinitbility.com' },
{ id: 2, name: 'aguidehub.com' },
{ id: 2, name: 'sortoutcode.com' },
];
setDomains(domains);
}, []);
return (
<View style={styles.container}>
{domains.map((user) => {
let keys = Object.keys(user);
return (
<Text style={styles.paragraph}>{keys.map((key) => {
return (
<Text>{`Key ${key}, value ${user[key]} \n`}</Text>
)
})}</Text>
)
})}
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
backgroundColor: '#ecf0f1',
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 18,
fontWeight: 'bold',
textAlign: 'center',
},
});
In the above program, we used the first map()
method to iterate and the second map()
method to iterate object keys it help us to get keys and values both from objects.
let’s check the output.
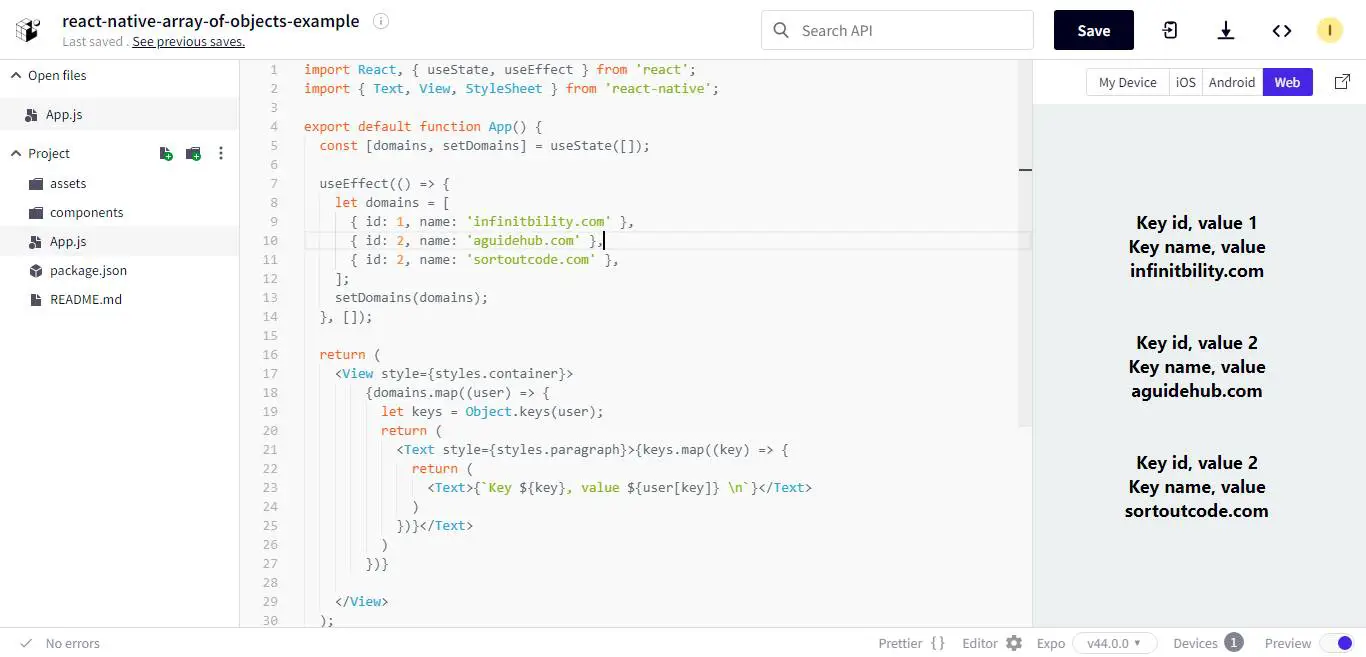
I hope it’s help you, All the best 👍.