Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
To append or push data into an array in react native, just use the push()
method it will add your data into an array as the last element.
Like the following example, we can push data into an array using the push()
method.
let arr = ["infinitbility","aguidehub"];
arr.push("sortoutcode");
console.log(arr); // ['infinitbility', 'aguidehub', 'sortoutcode']
Today, I’m going to show you How do i push data into an array in react native, as above mentioned here, I’m going to use the push()
method for adding new elements into the array.
Let’s start today’s tutorial how do you push data into an array in react native?
In this example, we will do
- Create a sample array state with sample data
- Add a new element into the array state using the
push()
method - Print array state in react native app screen
Let’s write code…
import React, { useState, useEffect } from 'react';
import { Text, View, StyleSheet } from 'react-native';
const App = () => {
const [arr, setArr] = useState([]);
useEffect(() => {
let newArr = ["infinitbility","aguidehub"];
newArr.push("sortoutcode");
setArr(newArr);
}, []);
return (
<View style={styles.container}>
<Text>{arr.toString()}</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
backgroundColor: '#ecf0f1',
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 18,
fontWeight: 'bold',
textAlign: 'center',
},
});
export default App;
Note: In the above code we have used the toString()
method because react native can’t show arr comma separated show we have converted to string then print on the screen
As mentioned above, we are taken the example of an array of strings, use the push()
to append an element, and printed it on the screen.
Let’s check the output.
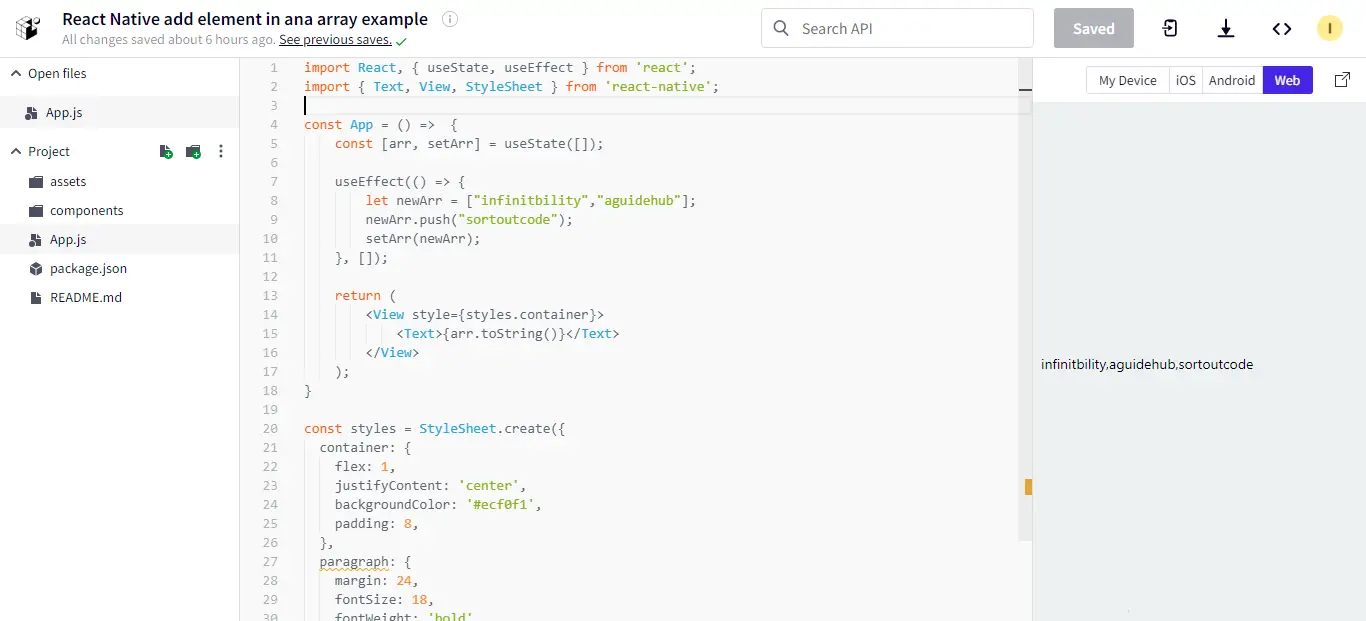
I hope it’s help you, All the best 👍.