Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
Today, we are going to learn how can we reverse an array without mutating in javascript, here we will use the javascript slice()
and reverse()
methods to do.
Table of contents
- Introduction
- Reverse array
- Reverse array without mutating
Let’s start today’s topic How to reverse an array without mutating in javascript?
Introduction
Let’s know what is a javascript slice and reverse method.
-
The
slice()
methodThe slice() method returns a shallow copy of a portion of an array into a new array object selected from start to end (end not included) where start and end represent the index of items in that array. The original array will not be modified.
– MDN
-
The
reverse()
methodThe reverse() method reverses an array in place. The first array element becomes the last, and the last array element becomes the first.
Reverse array
Here, we will use the array reverse method to do a reverse array with mutating and understand, what mutating means.
const array1 = ['one', 'two', 'three'];
console.log('array1:', array1);
// expected output: "array1:" Array ["one", "two", "three"]
const reversed = array1.reverse();
console.log('reversed:', reversed);
// expected output: "reversed:" Array ["three", "two", "one"]
// Careful: reverse is destructive -- it changes the original array.
console.log('array1:', array1);
// expected output: "array1:" Array ["three", "two", "one"]
When you read the above example, it’s reversing the original array also let’s see the output.
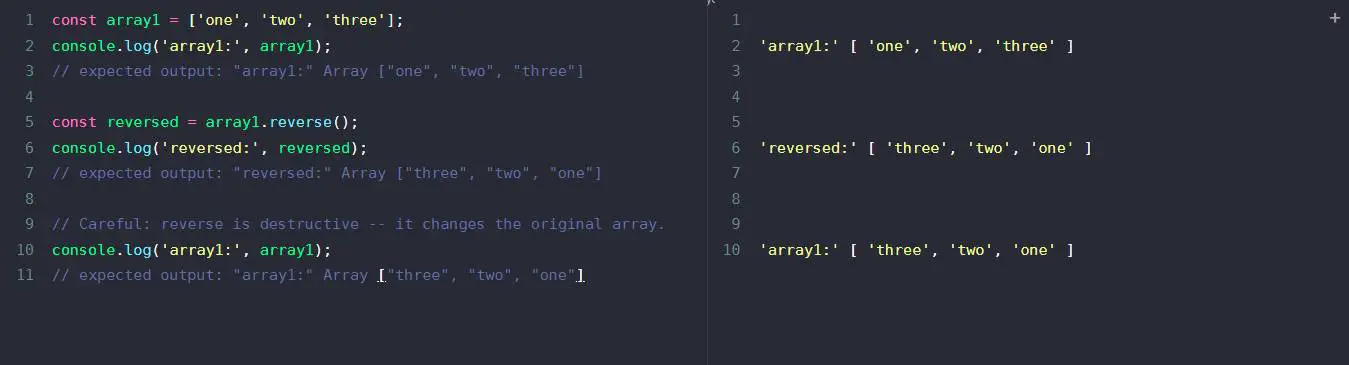
Reverse array without mutating
To handle, the mutating issue we have a lot’s ways but here we will see only two ways.
slice()
method- Spread operator ( … )
slice()
method
const array1 = ['one', 'two', 'three'];
console.log('array1:', array1);
// expected output: "array1:" Array ["one", "two", "three"]
const reversed = array1.slice().reverse();
console.log('reversed:', reversed);
// expected output: "reversed:" Array ["three", "two", "one"]
console.log('array1:', array1);
// expected output: 'array1:' [ 'one', 'two', 'three' ]
Here, we use the slice()
method to handle the mutating, let’s see the output.
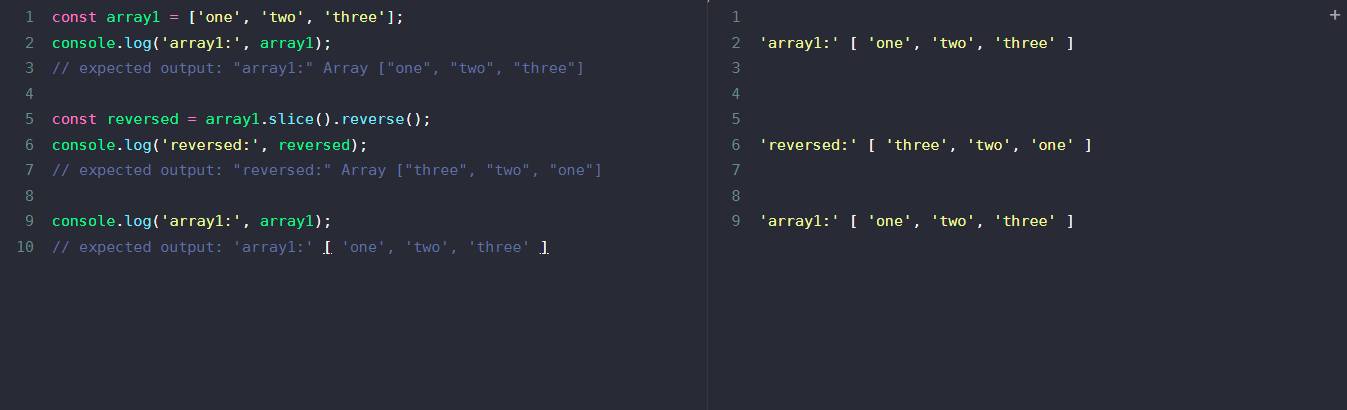
Spread operator ( … )
const array1 = ['one', 'two', 'three'];
console.log('array1:', array1);
// expected output: "array1:" Array ["one", "two", "three"]
const reversed = [...array1].reverse();
console.log('reversed:', reversed);
// expected output: "reversed:" Array ["three", "two", "one"]
console.log('array1:', array1);
// expected output: 'array1:' [ 'one', 'two', 'three' ]
Here, we are use spread operator ( ... )
method to handle the mutating, let’s see the output.
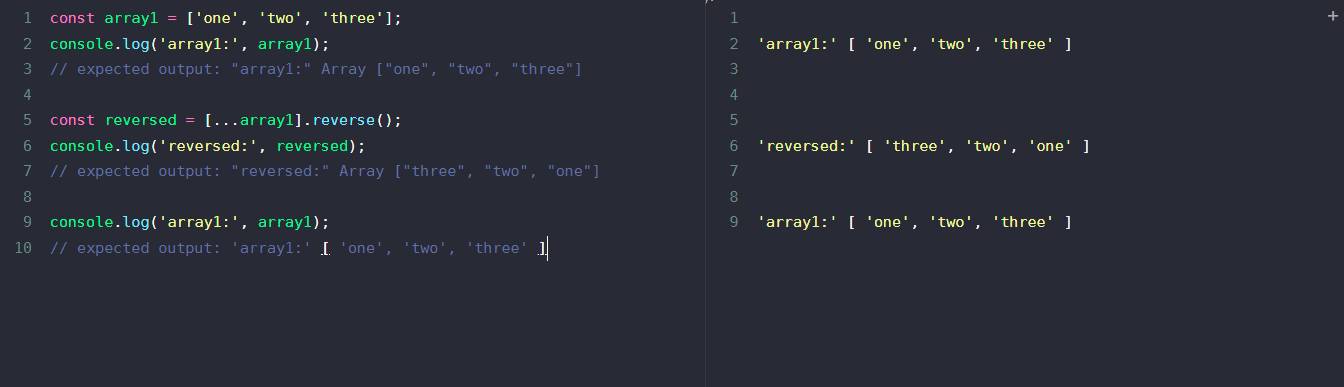
All the best 👍.