Hi Friends đź‘‹,
Welcome To Infinitbility! ❤️
To subtract two dates in moment js, just use the moment()
and diff()
method to subtract dates and get the difference in milliseconds ( You can specify your measurement unit like seconds, minutes, hours, days months, or years ).
Just import a moment in your file and use moment()
to create a moment date after you can use the diff()
method like the following example.
var a = moment([2007, 0, 29]);
var b = moment([2007, 0, 28]);
a.diff(b) // 86400000
Today, I’m going to show How do I subtract two dates in moment js, here I will use the momentjs common method moment()
to create the moment date object and the diff()
method to get the difference.
Let’s start the today’s tutorial How do you subtract two dates in moment js?
Table of content
- Installation
- Example in reactjs
Installation
Use the below installation command as per your package manager, moment support npm, Yarn, NuGet, spm, and meteor.
npm install moment --save # npm
yarn add moment # Yarn
Install-Package Moment.js # NuGet
spm install moment --save # spm
meteor add momentjs:moment # meteor
Example in reactjs
In the following example, we are going to do
- import the moment package
- example of 24-hour format time
HH:mm:ss
- example of 12-hour format time
h:mm:ss a
let’s write the code.
import moment from "moment";
function subtractDates() {
var date1 = moment('2016-10-08 10:29:23');
var date2 = moment('2021-10-08 11:06:55');
// milliseconds
date2.diff(date1); // 157768652000
// seconds
console.log(date2.diff(date1, 'seconds')) // 157768652
// minutes
console.log(date2.diff(date1, 'minutes')) // 2629477
// hours
console.log(date2.diff(date1, 'hours')) // 43824
// days
console.log(date2.diff(date1, 'days')) // 1826
// weeks
console.log(date2.diff(date1, 'weeks')) // 260
// months
console.log(date2.diff(date1, 'months')) // 60
// years
console.log(date2.diff(date1, 'years')) // 5
}
function App() {
subtractDates()
return (
<div>
{/* example of 24 hour format time `HH:mm:ss` */}
{moment().format("HH:mm:ss")}
</div>
);
}
export default App;
Note: By default, moment#diff will truncate the result to zero decimal places, returning an integer. If you want a floating point number, pass true as the third argument.
var a = moment([2008, 9]);
var b = moment([2007, 0]);
a.diff(b, 'years'); // 1
a.diff(b, 'years', true); // 1.75
In the above program, we call the moment()
and diff()
methods to subtract dates and get differences in years, months, weeks, days, hours, minutes, and seconds.
let’s check the output.
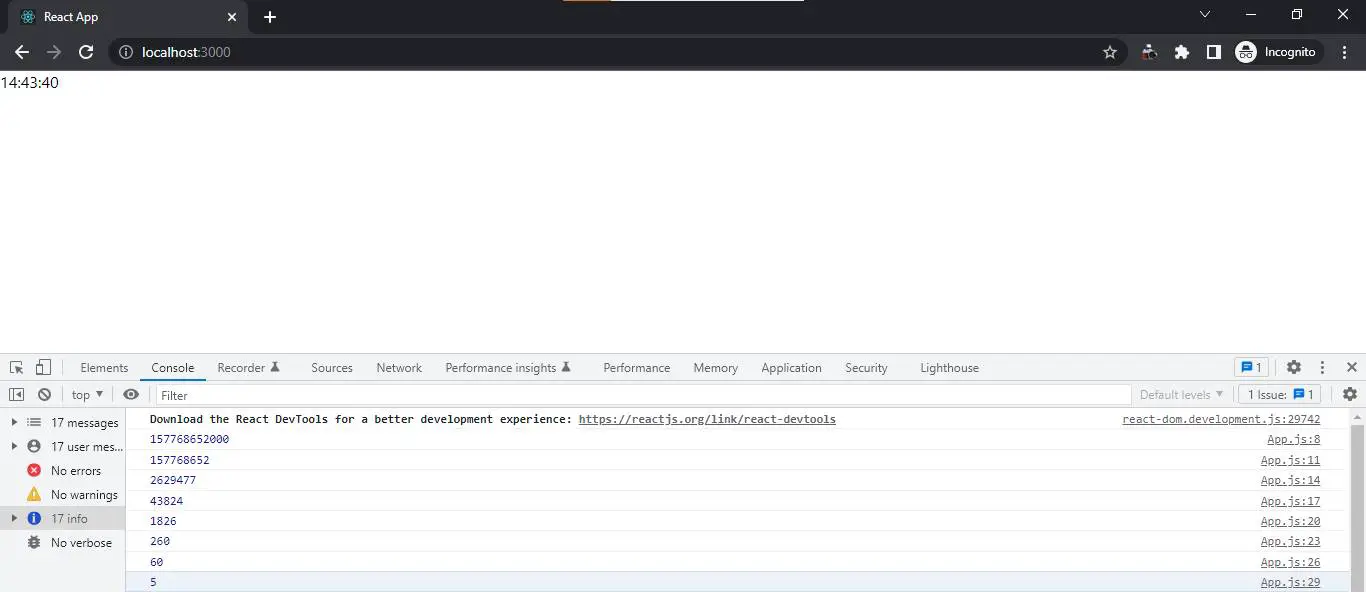
I hope it’s help you, All the best 👍.