Hello Friends đź‘‹,
Welcome To Infinitbility! ❤️
To get the current year in react native has the new Date().getFullYear()
method which will return every time the current year in number datatype.
we have to just call new Date().getFullYear()
and it will check the current date and return the current year based on the date.
let year = new Date().getFullYear();
console.log(year); // 2022
Now, we know how to get the current year in react native and the way to hold in a variable.
React native has a new Date()
object which is similar to javascript and the date object provides the getFullYear()
method which will return the year store in the new date object.
Let see how we can do in react native
import * as React from 'react';
import { Text, View, StyleSheet } from 'react-native';
export default function App() {
return (
<View style={styles.container}>
<Text style={styles.paragraph}>{new Date().getFullYear()}</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
backgroundColor: '#ecf0f1',
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 18,
fontWeight: 'bold',
textAlign: 'center',
},
});
Output
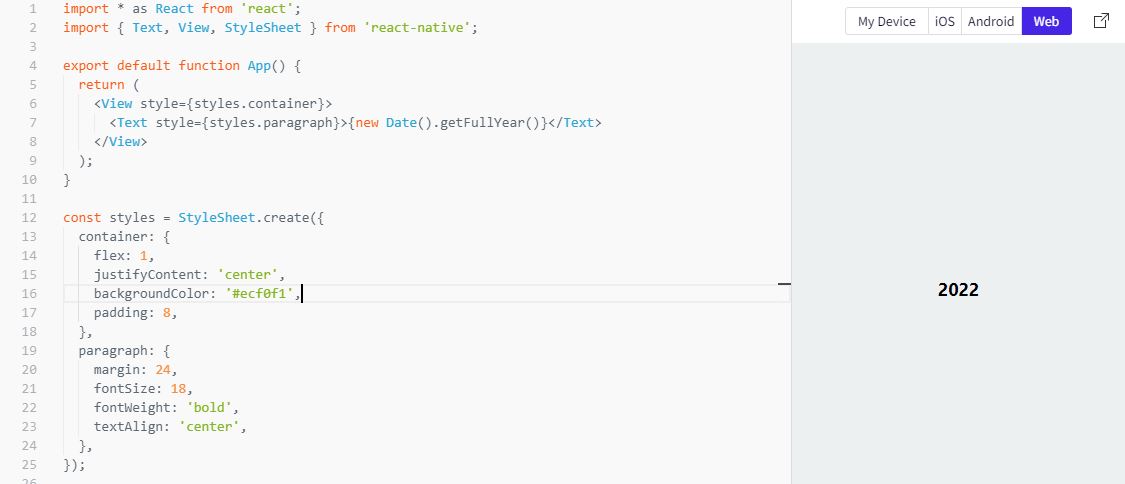
Thanks for reading…